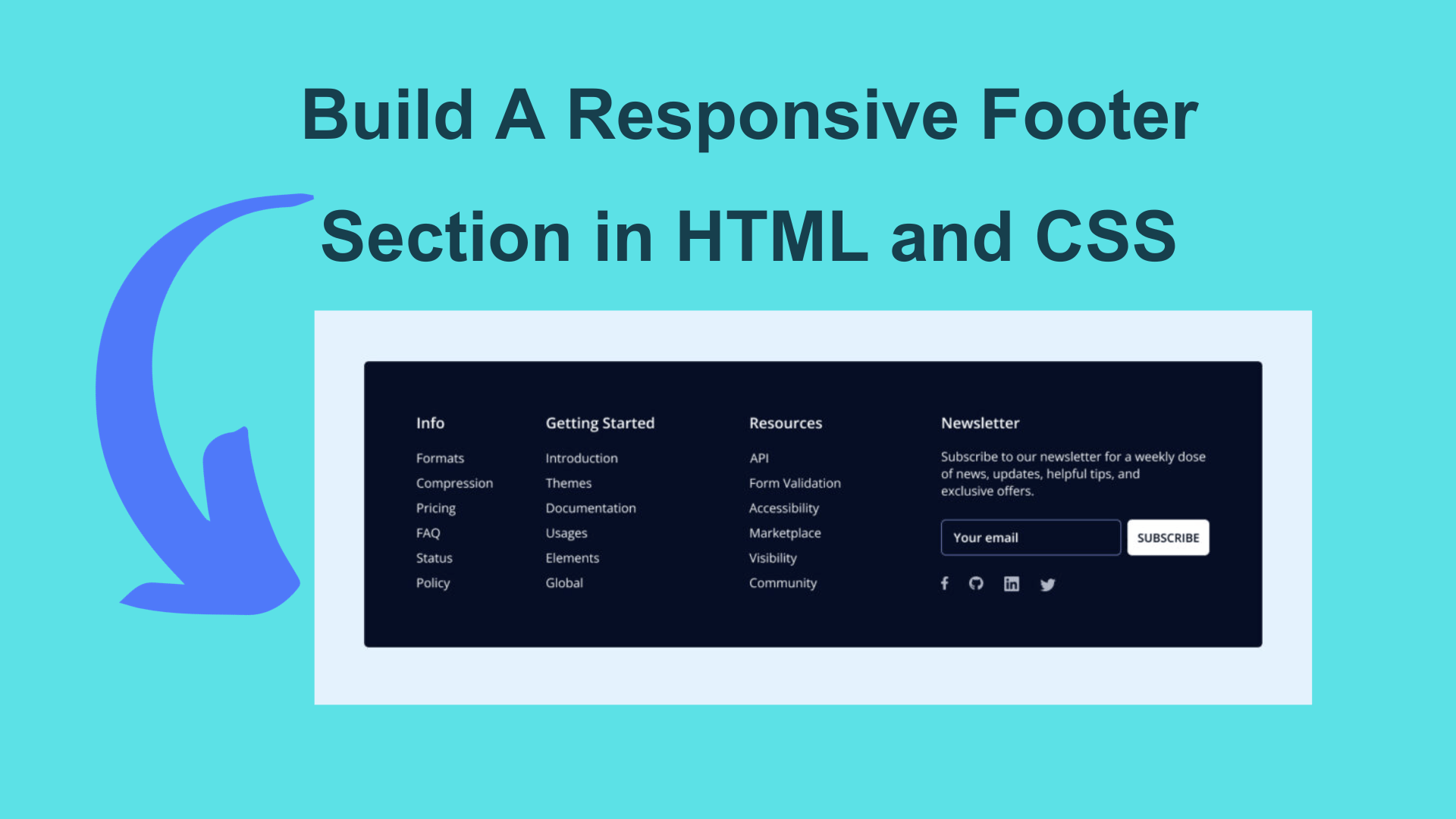
Build A Responsive Footer Section in HTML and CSS
Nearly every website you’ve visited likely has footers. At the bottom of every page, a website’s footer is an essential component. Typically, it includes copyright information and links to key sites.
I’ll demonstrate how to create a responsive footer with HTML and CSS in this blog article. It’s a straightforward yet excellent project for beginners that will show you how to use HTML to set up a footer layout and CSS to give it a fashionable, responsive look.
Common HTML elements like footer, h4, ul, li, a, and button will be utilized to construct a footer, along with some fundamental CSS attributes to design and make the footer responsive. Therefore, following along and comprehending the codes shouldn’t be a problem for you.
Using HTML and CSS, Create a Responsive Footer
These straightforward step-by-step tutorials will show you how to design a responsive footer using HTML and CSS:
Make a folder first with whatever name you choose. Create the appropriate files within it after that.
As the primary file, make a file named index.html.
For the CSS code, make a file named style.css.
Add the following HTML codes to your index.html file to get started: In order to develop our footer layout, this code comprises fundamental HTML markup with several semantic elements like footer, h4, form, input, p, li, and buttons.
- HTML FILE
<!DOCTYPE html>
<!-- Coding By CodingNepal - www.codingnepalweb.com -->
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Responsive Footer HTML and CSS | CodingNepal</title>
<!-- Fonts Links For Icon -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.2/css/all.min.css" />
<link rel="stylesheet" href="style.css" />
</head>
<body>
<section class="footer">
<div class="footer-row">
<div class="footer-col">
<h4>Info</h4>
<ul class="links">
<li><a href="#">About Us</a></li>
<li><a href="#">Compressions</a></li>
<li><a href="#">Customers</a></li>
<li><a href="#">Service</a></li>
<li><a href="#">Collection</a></li>
</ul>
</div>
<div class="footer-col">
<h4>Explore</h4>
<ul class="links">
<li><a href="#">Free Designs</a></li>
<li><a href="#">Latest Designs</a></li>
<li><a href="#">Themes</a></li>
<li><a href="#">Popular Designs</a></li>
<li><a href="#">Art Skills</a></li>
<li><a href="#">New Uploads</a></li>
</ul>
</div>
<div class="footer-col">
<h4>Legal</h4>
<ul class="links">
<li><a href="#">Customer Agreement</a></li>
<li><a href="#">Privacy Policy</a></li>
<li><a href="#">GDPR</a></li>
<li><a href="#">Security</a></li>
<li><a href="#">Testimonials</a></li>
<li><a href="#">Media Kit</a></li>
</ul>
</div>
<div class="footer-col">
<h4>Newsletter</h4>
<p>
Subscribe to our newsletter for a weekly dose
of news, updates, helpful tips, and
exclusive offers.
</p>
<form action="#">
<input type="text" placeholder="Your email" required>
<button type="submit">SUBSCRIBE</button>
</form>
<div class="icons">
<i class="fa-brands fa-facebook-f"></i>
<i class="fa-brands fa-twitter"></i>
<i class="fa-brands fa-linkedin"></i>
<i class="fa-brands fa-github"></i>
</div>
</div>
</div>
</section>
</body>
</html>
Then, include the subsequent CSS codes in your style.Use a CSS file to create a stylish and functional footer. To give your footer a unique touch, try with various CSS attributes like colors, fonts, and backgrounds. You can now view your footer design if you open the website in your browser.
- CSS FILE
/* Importing Google font - Open Sans */
@import url('https://fonts.googleapis.com/css2?family=Open+Sans:wght@300;400;500;600;700&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Open Sans', sans-serif;
}
.footer {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
max-width: 1280px;
width: 95%;
background: #10182F;
border-radius: 6px;
}
.footer .footer-row {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
gap: 3.5rem;
padding: 60px;
}
.footer-row .footer-col h4 {
color: #fff;
font-size: 1.2rem;
font-weight: 400;
}
.footer-col .links {
margin-top: 20px;
}
.footer-col .links li {
list-style: none;
margin-bottom: 10px;
}
.footer-col .links li a {
text-decoration: none;
color: #bfbfbf;
}
.footer-col .links li a:hover {
color: #fff;
}
.footer-col p {
margin: 20px 0;
color: #bfbfbf;
max-width: 300px;
}
.footer-col form {
display: flex;
gap: 5px;
}
.footer-col input {
height: 40px;
border-radius: 6px;
background: none;
width: 100%;
outline: none;
border: 1px solid #7489C6 ;
caret-color: #fff;
color: #fff;
padding-left: 10px;
}
.footer-col input::placeholder {
color: #ccc;
}
.footer-col form button {
background: #fff;
outline: none;
border: none;
padding: 10px 15px;
border-radius: 6px;
cursor: pointer;
font-weight: 500;
transition: 0.2s ease;
}
.footer-col form button:hover {
background: #cecccc;
}
.footer-col .icons {
display: flex;
margin-top: 30px;
gap: 30px;
cursor: pointer;
}
.footer-col .icons i {
color: #afb6c7;
}
.footer-col .icons i:hover {
color: #fff;
}
@media (max-width: 768px) {
.footer {
position: relative;
bottom: 0;
left: 0;
transform: none;
width: 100%;
border-radius: 0;
}
.footer .footer-row {
padding: 20px;
gap: 1rem;
}
.footer-col form {
display: block;
}
.footer-col form :where(input, button) {
width: 100%;
}
.footer-col form button {
margin: 10px 0 0 0;
}
}
Conclusion
In conclusion, building a nice footer using HTML and CSS is an easy yet worthwhile online project for a novice. Your newfound abilities may be applied to the creation of a number of website elements, including login forms, navigation bars, home pages, and cards.
If you encounter any problems while creating your footer, you can download the source code files for this footer project for free by clicking the Download button. Additionally, you can view a live demo of it by clicking the View Live button.
[…] Build A Responsive Footer Section in HTML and CSS […]