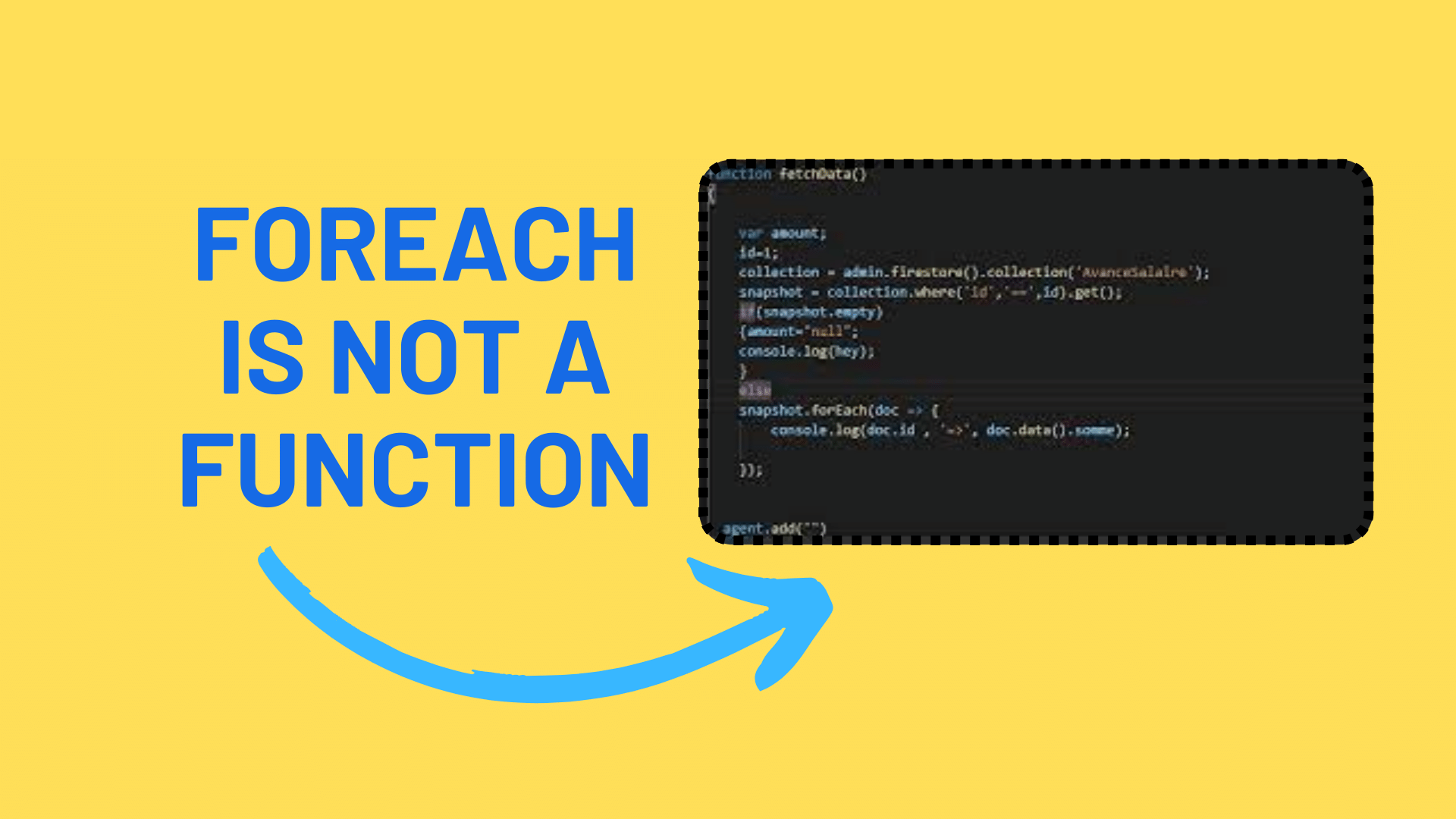
How to Handle ‘ForEach is not a Function’ Errors in Your Code
When working with JavaScript, you may encounter the “ForEach is not a function” error, especially when dealing with arrays or array-like objects. In this blog post, we will explore this error in detail and provide solutions to resolve it. We’ll also discuss common scenarios where this error can occur and how to avoid it.
Understanding the Error:
Let’s start by examining the error message itself:
VM384:53 Uncaught TypeError: parent.children.forEach is not a function
This error occurs when you attempt to use the forEach
method on an object that doesn’t support it. In this specific case, parent.children
is the culprit. It’s important to note that parent.children
is not a regular JavaScript array; instead, it’s an HTMLCollection or a NodeList, which are array-like objects but lack the full array functionality.
Common Scenarios:
- Using
parent.children
: As demonstrated in the code snippet below, attempting to useforEach
directly onparent.children
will result in the error:
const parent = this.el.parentElement;
parent.children.forEach(child => {
console.log(child);
});
- Using
element.siblings
: A similar issue arises when trying to iterate through siblings usingelement.siblings
, as shown below:
element.siblings.forEach(sibling => {
console.log(sibling);
});
- Case Sensitivity: JavaScript is case-sensitive, so make sure you use the correct capitalization when calling
forEach
. It should beforEach
, notforeach
.
Solutions:
Now that we understand the problem, let’s explore solutions to resolve the “ForEach is not a function” error.
1. Indirect Invocation of forEach
:
To work with HTMLCollections or NodeLists, you can indirectly invoke forEach
by borrowing it from the Array.prototype
. Here’s how to do it:
const parent = this.el.parentElement;
Array.prototype.forEach.call(parent.children, child => {
console.log(child);
});
2. Using the Iterable Protocol:
HTMLCollections and NodeLists are iterable, which means you can use the iterable protocol to loop through their elements. Here are two ways to achieve this:
a. Using the Spread Operator:
const parent = this.el.parentElement;
[...parent.children].forEach(child => {
console.log(child);
});
b. Using the for...of
Loop:
const parent = this.el.parentElement;
for (const child of parent.children) {
console.log(child);
}
3. Converting to an Array:
You can convert the HTMLCollection or NodeList to a regular JavaScript array and then use forEach
without any issues. Here’s how to do it:
const parent = this.el.parentElement;
const childrenArray = Array.from(parent.children);
childrenArray.forEach(child => {
console.log(child);
});
4. Using a Traditional for
Loop:
If you prefer a more traditional approach, you can use a for
loop to iterate through the elements:
const parent = this.el.parentElement;
const children = parent.children;
for (let i = 0; i < children.length; i++) {
const child = children[i];
console.log(child);
}
Conclusion:
The “ForEach is not a function” error is a common issue when working with HTMLCollections and NodeLists in JavaScript. By understanding the nature of these array-like objects and using the provided solutions, you can successfully iterate through their elements and avoid this error. Remember to pay attention to case sensitivity and choose the method that best fits your coding style and project requirements.
By following the tips and solutions outlined in this blog post, you can handle the “ForEach is not a function” error with confidence and write more robust JavaScript code. Happy coding!
[…] How to Handle ‘ForEach is not a Function’ Errors in Your Code […]