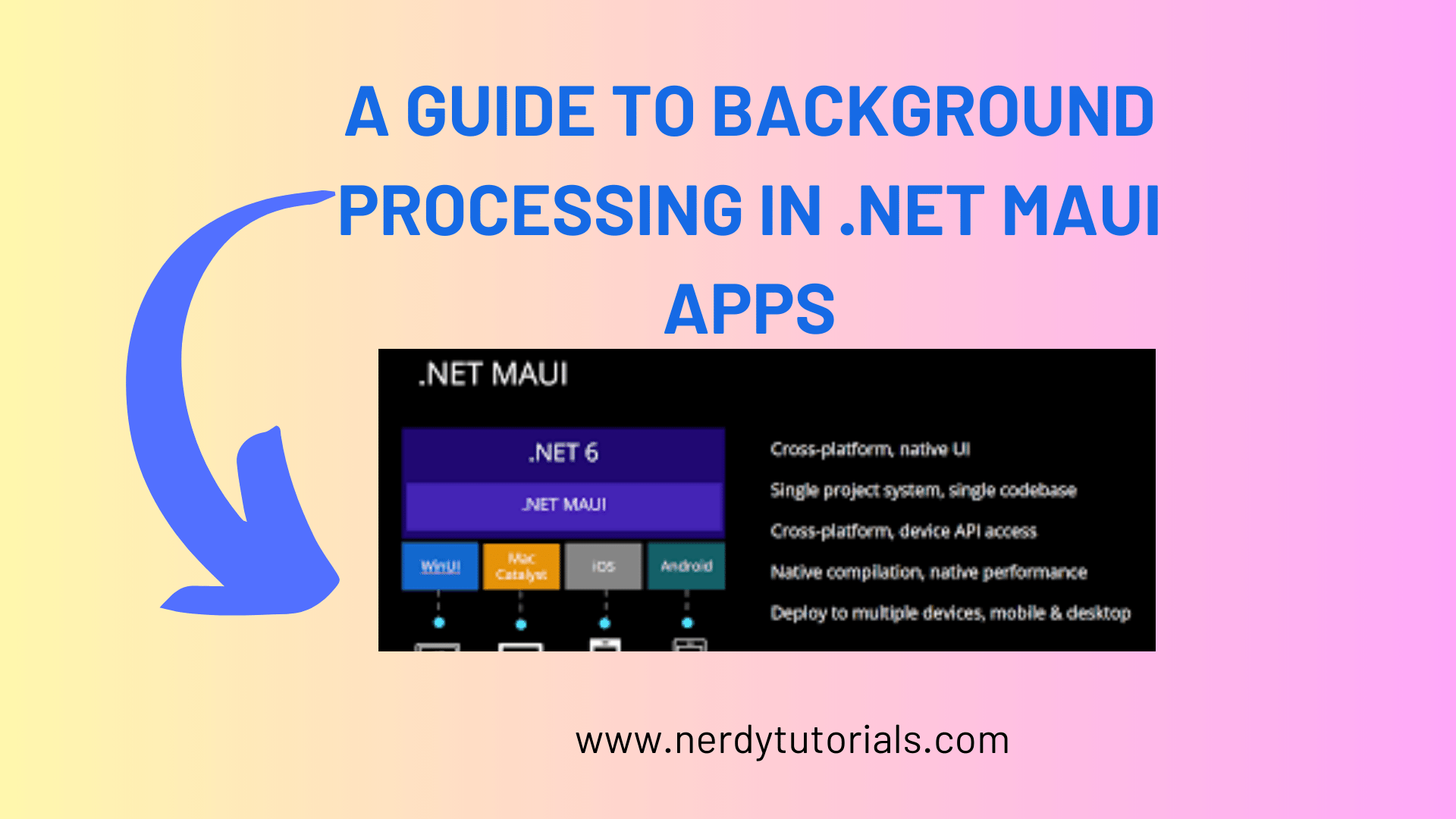
How to Background Processing in .NET MAUI Apps
In the realm of mobile app development, there are times when you need your application to perform tasks or continue functioning even when it’s not in the foreground. This is particularly true for scenarios like playing music in the background while the user interacts with other apps or device functionalities. In this guide, we’ll explore how to achieve background processing in .NET MAUI apps, focusing on the Android platform.
Background Music Playback in .NET MAUI
Our scenario involves a .NET MAUI app running on an Android device, and we want to ensure that the music playback continues even when the app is not in focus. One common approach suggested by developers is to use an Android service. However, there are nuances to consider, especially if your app needs to perform other tasks such as making API calls or calculations while keeping the music stream alive.
You Might Like This :
- A Guide to Effective .NET 7 Program.cs Configuration
- Advanced Argument Parsing Techniques in .NET 6 Console Apps
- Resolving Object or Property Not Found Issues in .NET Framework 4.8
- Full Stack Development vs Specialized .NET Development
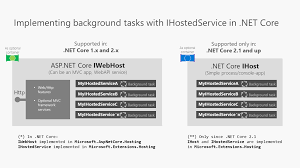
Using Android Services
As mentioned by Jason in the initial question, one of the fundamental mechanisms Android provides for running tasks in the background is through services. Services are components that run in the background independently of the main application, allowing you to perform tasks like playing music or updating data.
To implement background music playback, you can create an Android service dedicated to this purpose. The service will be responsible for managing the music stream, ensuring it continues without interruption even when the app is paused or not in focus.
Here’s a simplified example of how you can create a background music service in your .NET MAUI app:
public class BackgroundMusicService : Service
{
// Your music playback logic goes here
public override IBinder OnBind(Intent intent)
{
// Return null since this is not a bound service
return null;
}
public override StartCommandResult OnStartCommand(Intent intent, StartCommandFlags flags, int startId)
{
// Start your music playback here
return StartCommandResult.Sticky;
}
public override void OnDestroy()
{
// Stop and release any resources when the service is destroyed
base.OnDestroy();
}
}
In this example, the BackgroundMusicService
class extends Service
and handles music playback within the OnStartCommand
method. You can tailor this service to meet your specific requirements, including managing the music stream, API calls, and calculations.
Handling App Lifecycle
While using a service is a common and recommended way to handle background tasks like music playback, it’s essential to understand the app’s lifecycle and how to interact with the service accordingly.
In your .NET MAUI app, you can override the OnPause
and OnResume
methods to manage the service when the app is paused or resumed:
protected override void OnPause()
{
// Pause or manage your service when the app is paused
base.OnPause();
}
protected override void OnResume()
{
// Resume your service when the app is resumed
base.OnResume();
}
These methods allow you to control the behavior of your background music service based on the app’s lifecycle events.
Considerations and Alternatives
While using an Android service is a robust and recommended approach, it’s essential to weigh the specific requirements of your app. If your app demands complex background processing, such as frequent API calls and calculations alongside music playback, you may need to design your service accordingly to handle these tasks efficiently.
Additionally, you can explore other Android-specific features like Foreground Services, which provide a way to give foreground-like priority to a service. This can be useful when your app requires consistent and high-priority background processing.
Conclusion
In this guide, we’ve explored how to achieve background processing in .NET MAUI apps, focusing on the Android platform. We’ve discussed the use of Android services to handle background music playback and provided a basic example to get you started. Remember to consider your app’s specific requirements and lifecycle events when implementing background processing to ensure a seamless user experience. With the right approach, you can create engaging and functional .NET MAUI apps that perform tasks efficiently, even when not in the foreground.
[…] A Guide to Background Processing in .NET MAUI Apps […]
[…] A Guide to Background Processing in .NET MAUI Apps […]