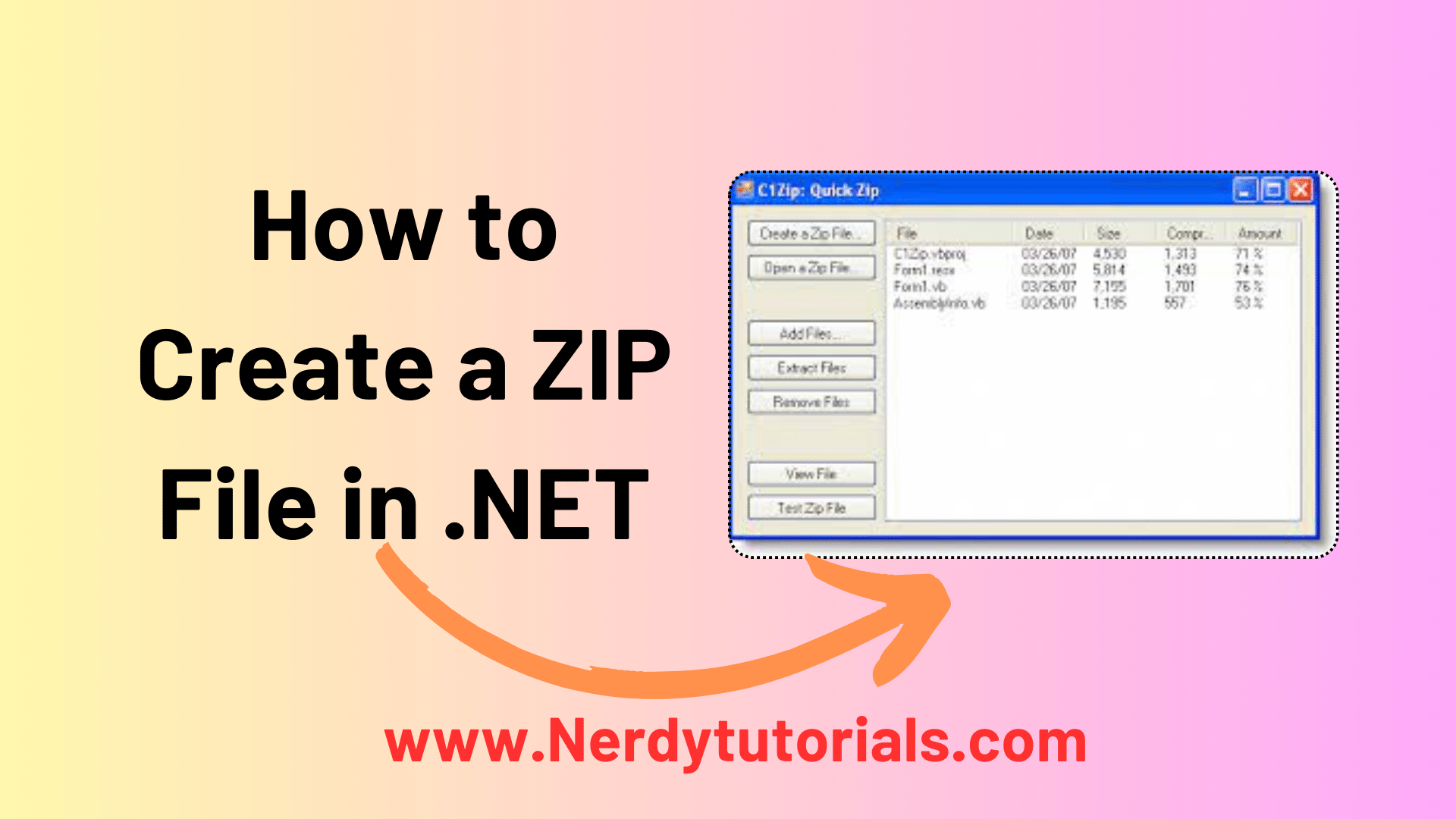
How to Create a ZIP File in .NET
In the world of software development, there often comes a time when you need to handle file operations efficiently. One common task is generating PDF files on the fly and then packaging them into a zip file for easy distribution. In this tutorial, we will explore how to achieve this seamlessly in .NET MVC using C#. We’ll delve into the use of in-memory byte arrays and the ZipArchive
class from System.IO.Compression
, eliminating the need to write temporary files to disk.
The Scenario
Imagine you are working on an MVC project, and your goal is to generate multiple PDF files dynamically (perhaps using a tool like wkhtmltopdf) and return them to the user as a zip file. Each PDF file is generated on the fly, and you don’t need to store them permanently. The typical code to return a single PDF file in ASP.NET MVC looks like this:
You Might Like This :
- Managing Environment Variables in a .NET Console Application
- Mastering .NET Development: Backend vs. Frontend
- .NET Array Transformation: From Data to String
- A Guide to Effective .NET 7 Program.cs Configuration
- Advanced Argument Parsing Techniques in .NET 6 Console Apps
File(pdfBytes, "application/pdf", "file_name")
However, you want to go a step further and create multiple PDF files on the fly, then bundle them into a zip archive and return it to the user. You might envision code like this:
ZipFile zip = new ZipFile();
foreach(var html in foundRawHTML)
{
// Generate PDF
// Append PDF to zip
}
return zip;
Unfortunately, this approach faces a couple of challenges:
ZipFile
andFile
classes are static and cannot be instantiated.- There’s no direct way to add a file to a zip archive in memory.
The Solution
To overcome these challenges, we can use in-memory byte arrays and the ZipArchive
class. Here’s a step-by-step guide on how to achieve this:
Step 1: Create a Helper Method
First, let’s create a helper method that takes a list of in-memory files and returns a byte array representing the zip archive:
public static byte[] GetZipArchive(List<InMemoryFile> files)
{
byte[] archiveFile;
using (var archiveStream = new MemoryStream())
{
using (var archive = new ZipArchive(archiveStream, ZipArchiveMode.Create, true))
{
foreach (var file in files)
{
var zipArchiveEntry = archive.CreateEntry(file.FileName, CompressionLevel.Fastest);
using (var zipStream = zipArchiveEntry.Open())
zipStream.Write(file.Content, 0, file.Content.Length);
}
}
archiveFile = archiveStream.ToArray();
}
return archiveFile;
}
Step 2: Define the InMemoryFile Class
Create a simple class to represent in-memory files:
public class InMemoryFile
{
public string FileName { get; set; }
public byte[] Content { get; set; }
}
Step 3: Generate PDF Files and Create the Zip Archive
Now, you can generate your PDF files on the fly, add them to the list of InMemoryFile
objects, and create the zip archive:
List<InMemoryFile> pdfFiles = new List<InMemoryFile>();
foreach (var html in foundRawHTML)
{
// Generate PDF from HTML (use wkhtmltopdf or your preferred tool)
byte[] pdfBytes = GeneratePdfFromHtml(html);
// Add the PDF to the list
pdfFiles.Add(new InMemoryFile
{
FileName = "document" + pdfFiles.Count + ".pdf",
Content = pdfBytes
});
}
// Create the zip archive
byte[] zipArchive = GetZipArchive(pdfFiles);
// Return the zip archive to the user
return File(zipArchive, "application/zip", "pdf_files.zip");
That’s it! You’ve efficiently generated PDF files on the fly, packaged them into a zip archive in memory, and returned the zip archive to the user.
Conclusion
In this tutorial, we’ve explored a practical approach to generate PDF files on the fly and bundle them into a zip archive without the need for temporary files on disk. Leveraging in-memory byte arrays and the ZipArchive
class in .NET allows you to efficiently handle file operations in your MVC projects. This solution enhances the user experience by providing a convenient way to download multiple PDF files in a single zip archive. Happy coding!
Remember to customize the code to fit your specific requirements and integrate it seamlessly into your .NET MVC project.
[…] How to Create a ZIP File in .NET […]
[…] How to Create a ZIP File in .NET […]