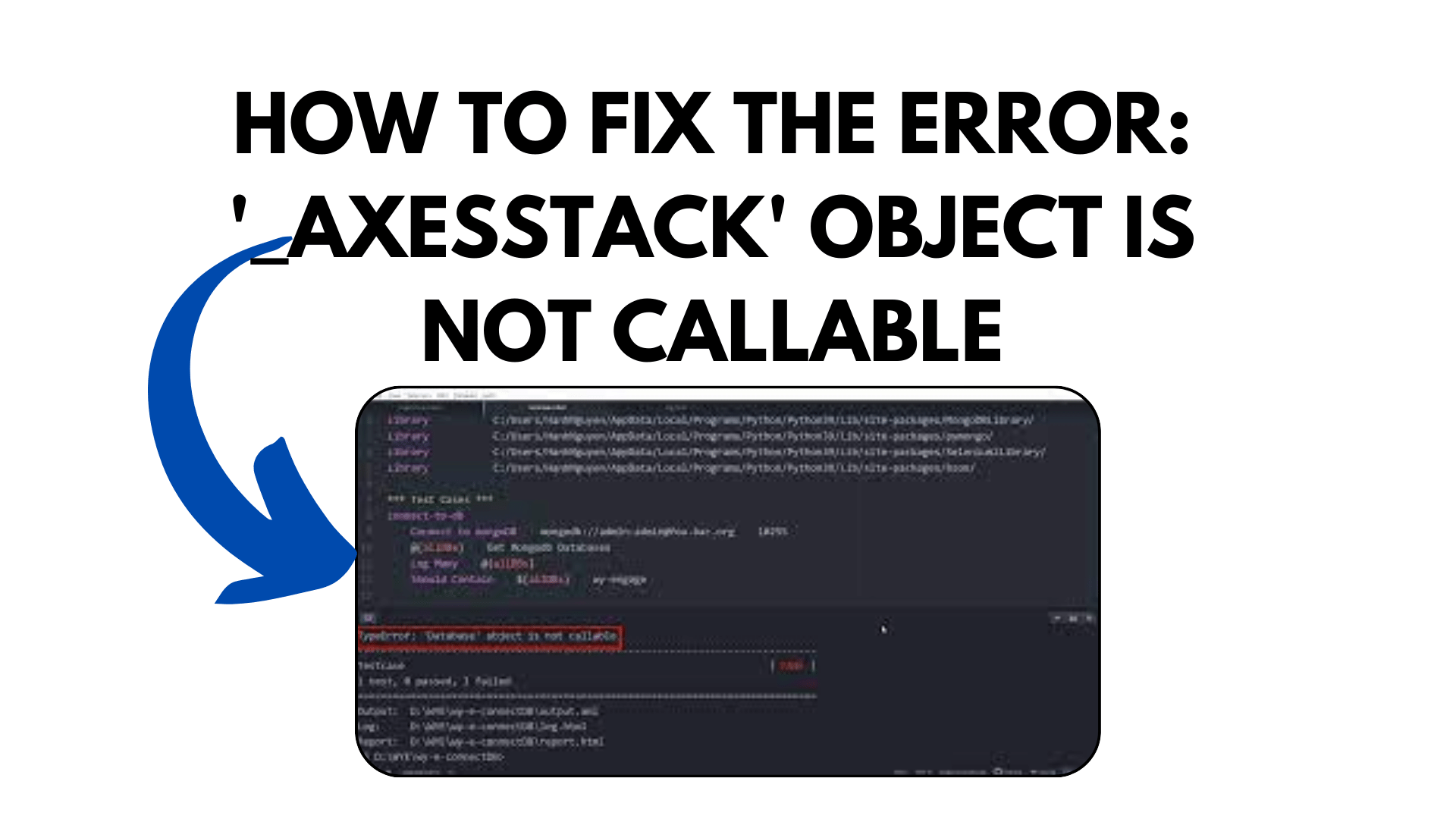
How to Fix the Error: ‘_axesstack’ Object is not Callable
Are you trying to visualize a directed acyclic unweighted matrix using NetworkX and encountering the error message “_AxesStack’ object is not callable”? This error can be frustrating, but it’s usually caused by a small mistake in your code. In this blog post, we’ll explore the possible reasons behind this error and provide you with a solution.
Understanding the Error
The error message “_AxesStack’ object is not callable” is typically related to a problem with the Matplotlib library. This error can occur when you are trying to draw a graph using NetworkX and Matplotlib, and it often indicates a compatibility issue between the two libraries.
The Code
Let’s start by examining the code you’ve provided:
import networkx as nx
import matplotlib.pyplot as plt
import matplotlib
matplotlib.use('TkAgg')
import numpy as np
A = np.array([[0,1,1,0],
[1,0,1,1],
[1,1,0,0],
[0,1,0,0]])
G = nx.from_numpy_array(A)
nx.draw(G, with_labels=True)
The code appears to be an attempt to create a graph using NetworkX and display it using Matplotlib. However, as you’ve encountered, there seems to be an issue with the nx.draw()
function.
Solution
The error you’re facing is likely due to a recent change in the NetworkX library. To resolve this issue, you should use the nx.draw_networkx()
function instead of nx.draw()
. Here’s the modified code:
import networkx as nx
import matplotlib.pyplot as plt
import matplotlib
matplotlib.use('TkAgg')
import numpy as np
A = np.array([[0,1,1,0],
[1,0,1,1],
[1,1,0,0],
[0,1,0,0]])
G = nx.from_numpy_array(A)
nx.draw_networkx(G, with_labels=True)
By making this simple change, you should be able to avoid the “_AxesStack’ object is not callable” error.
Version Compatibility
In your provided details, you mentioned that you are using NetworkX version 2.8.4 and Matplotlib version 3.6.0, matching the versions mentioned in the tutorial. It’s important to note that software libraries are continually evolving, and sometimes, there can be compatibility issues between different versions. In this case, the nx.draw()
function may have been updated in NetworkX version 3.0, and it’s no longer callable.
To ensure smooth operation, consider updating both NetworkX and Matplotlib to their latest versions. This may require using pip
to install the most recent releases:
pip install --upgrade networkx matplotlib
Viewing the Graph
You also mentioned that when you run the code in PyCharm, you can see that the program runs successfully, but no graph is displayed. This issue could be related to your environment setup. To visualize the graph in PyCharm, make sure that Matplotlib can display the graph properly. This might involve configuring Matplotlib to work with your PyCharm environment.
Conclusion
In this blog post, we explored the error “_AxesStack’ object is not callable” in the context of using NetworkX and Matplotlib to visualize a graph. By updating your code to use the nx.draw_networkx()
function and ensuring compatibility between your library versions, you can resolve this error and successfully visualize your graphs. If you encounter any further issues or have additional questions, feel free to ask for assistance. Happy coding!