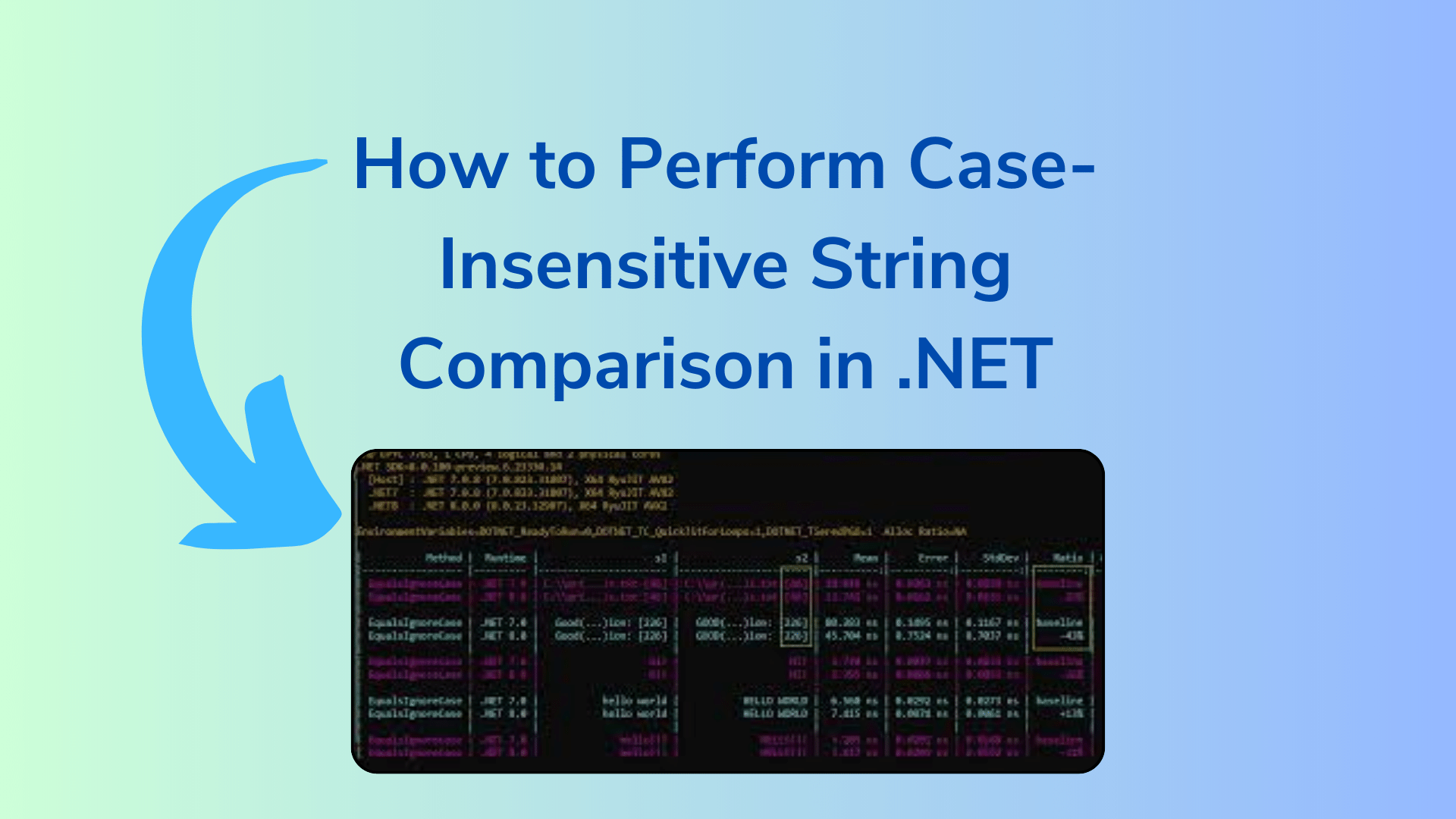
How to Perform Case-Insensitive String Comparison in .NET
In the world of programming, string comparison is a common task. Often, you need to compare two strings, but it’s not always as straightforward as it seems. One frequent requirement is performing case-insensitive string comparison. In this blog post, we’ll explore how to achieve this in .NET.
The Scenario: Let’s begin with a real-world scenario. Imagine you have a collection of user data, and you want to determine if a user is enrolled based on their username. You have been given advice on how to perform a case-insensitive comparison, but you are encountering issues. Here’s the code snippet in question:
drUser["Enrolled"] = (enrolledUsers.FindIndex(x => x.Username.Equals((string)drUser["Username"], StringComparison.OrdinalIgnoreCase)));
The Trouble: The code above should ideally set the “Enrolled” field to true if a match is found between the usernames, regardless of the case. However, it’s returning unexpected results, marking enrolled users as unenrolled and vice versa.
You Might Like This :
- .NET XML Processing Made Easy: Reading XML Files
- How to Handle “.NET Location Not Found” in Your .NET Projects
- How to Retrieve the Current Directory in .NET
- Resolving Object or Property Not Found Issues in .NET Framework 4.8
Let’s dig into the solutions and understand what might be causing this issue.
Solution 1:
String.Compare One of the recommended ways to perform case-insensitive string comparison in .NET is to use the String.Compare
method. Here’s how you can modify your code:
drUser["Enrolled"] = (enrolledUsers.FindIndex(x => String.Compare(x.Username, (string)drUser["Username"], StringComparison.OrdinalIgnoreCase) == 0));
This code compares the two strings using the String.Compare
method and checks if the result is equal to 0, indicating a match.
Solution 2:
String.Equals Another approach is to use the String.Equals
method with a StringComparison parameter. Here’s how you can do it:
drUser["Enrolled"] = (enrolledUsers.FindIndex(x => x.Username.Equals((string)drUser["Username"], StringComparison.OrdinalIgnoreCase)));
This code is similar to your initial attempt but should work as expected.
Solution 3: StringComparison.CurrentCultureIgnoreCase If you want to use the String.Equals
method and prefer a different StringComparison option, you can try StringComparison.CurrentCultureIgnoreCase
:
drUser["Enrolled"] = (enrolledUsers.FindIndex(x => x.Username.Equals((string)drUser["Username"], StringComparison.CurrentCultureIgnoreCase)));
Conclusion:
In this blog post, we explored how to perform case-insensitive string comparison in .NET. We discussed two primary methods, String.Compare
and String.Equals
, both of which can be used with the StringComparison.OrdinalIgnoreCase
option to achieve our goal.
Remember that choosing the right comparison method depends on your specific requirements. It’s essential to understand the behavior of each approach and test them thoroughly to ensure they meet your needs.
String comparison is a fundamental operation in programming, and knowing how to perform it accurately, especially when dealing with case sensitivity, is a valuable skill for any .NET developer
[…] How to Perform Case-Insensitive String Comparison in .NET […]