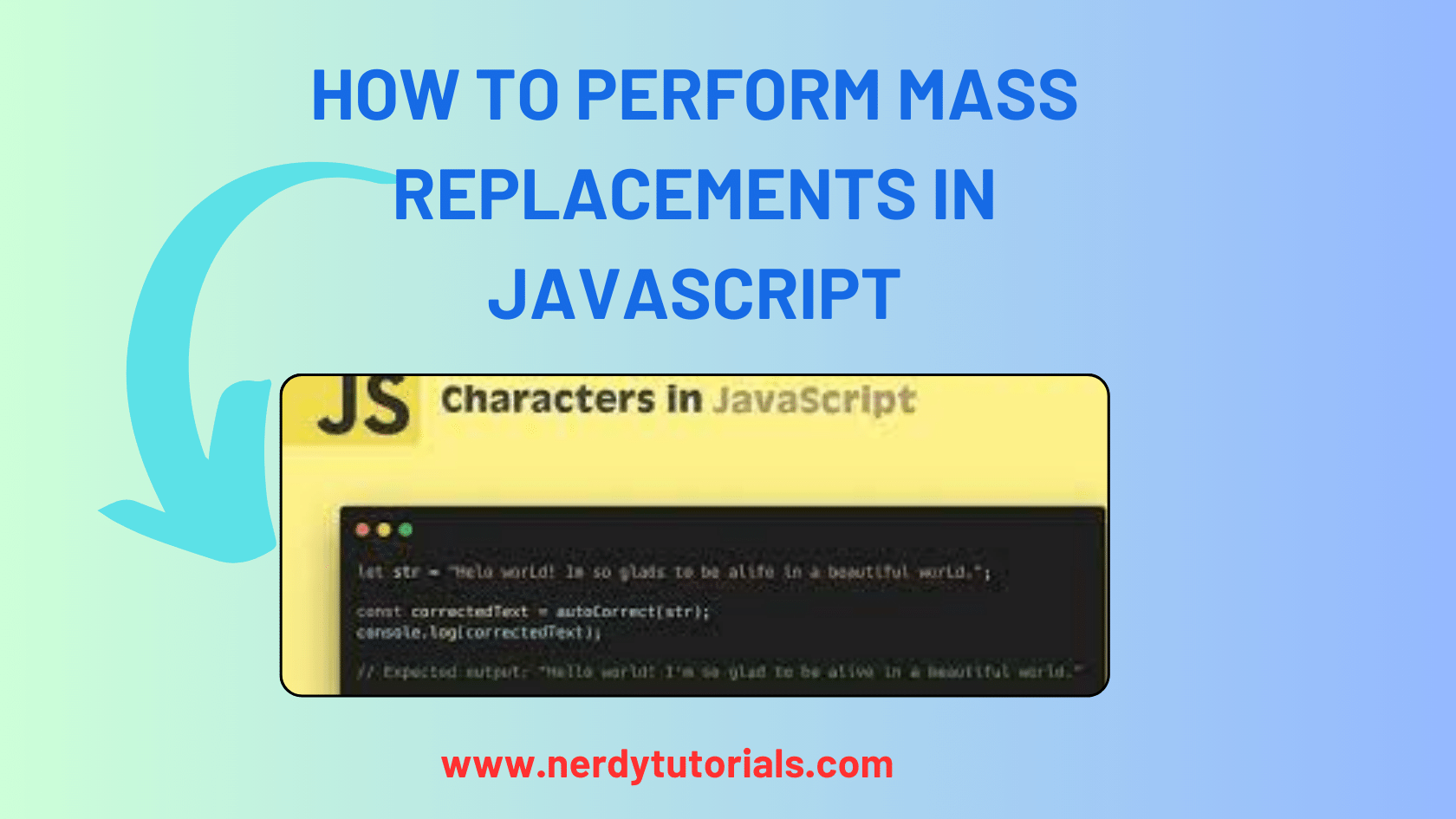
How to Perform Mass Replacements in JavaScript
When working with strings in JavaScript, you might encounter situations where you need to replace multiple characters or substrings within a string. For instance, you may want to replace all occurrences of one character with another or remove specific characters altogether. In this tutorial, we’ll explore different methods for performing mass replacements in JavaScript.
The Problem
Let’s start with a practical problem. Imagine you have a string containing special characters like underscores (_) and hash symbols (#), and you need to manipulate the string by replacing underscores with spaces and removing hash symbols. Here’s an example string:
var string = '#Please send_an_information_pack_to_the_following_address:';
You may have attempted something like this:
string.replace('#','').replace('_', ' ');
While this approach does work, it involves chaining multiple replace
methods, which might not be the most elegant solution. Is there a more efficient way to achieve the same result in just one step? Let’s explore some options.
You Might Like This :
- Solving Form Validation Challenges with HTML, CSS, and JavaScript
- How to Resolve ‘MockReturnValue is not a Function’ Errors in Unit Testing
- TypeError: Indexof is not a function – Troubleshooting JavaScript Errors
- How to Fix ‘Includes is not a function’ Errors
Using Regular Expressions
One of the most flexible ways to perform mass replacements in JavaScript is by using regular expressions. You can create a regular expression pattern that matches all the characters you want to replace and specify the replacement values.
Here’s how you can achieve the desired replacement in a single step:
var result = string.replace(/#|_/g, '');
In this regular expression, the #|_
pattern matches either a hash symbol or an underscore, and the g
flag ensures that all matching characters in the string are replaced with an empty string.
Replacing with Custom Values
If you need to replace different characters with distinct values, you can create a mapping object and use a callback function within the replace
method. Here’s an example:
function replaceChars(match) {
var replacements = {
'#': '', // Replace '#' with an empty string
'_': ' ' // Replace '_' with a space
};
return replacements[match];
}
var result = string.replace(/#|_/g, replaceChars);
In this case, the replaceChars
function is used as a callback for each matched character, and it returns the corresponding replacement value from the replacements
object.
Conclusion
Performing mass replacements in JavaScript can be accomplished using regular expressions and custom callback functions. Whether you need to replace multiple characters with a common value or replace them individually, these techniques provide efficient solutions for manipulating strings.
Remember that regular expressions offer great flexibility, allowing you to target various patterns within a string. Experiment with different regular expressions and callback functions to suit your specific needs when performing mass replacements in JavaScript.
That’s it for this tutorial! We hope you found it helpful in tackling string manipulation tasks in your JavaScript projects. If you have any questions or would like to explore more JavaScript topics, feel free to leave a comment or visit our website for additional tutorials and coding tips.Happy coding!
[…] How to Perform Mass Replacements in JavaScript […]