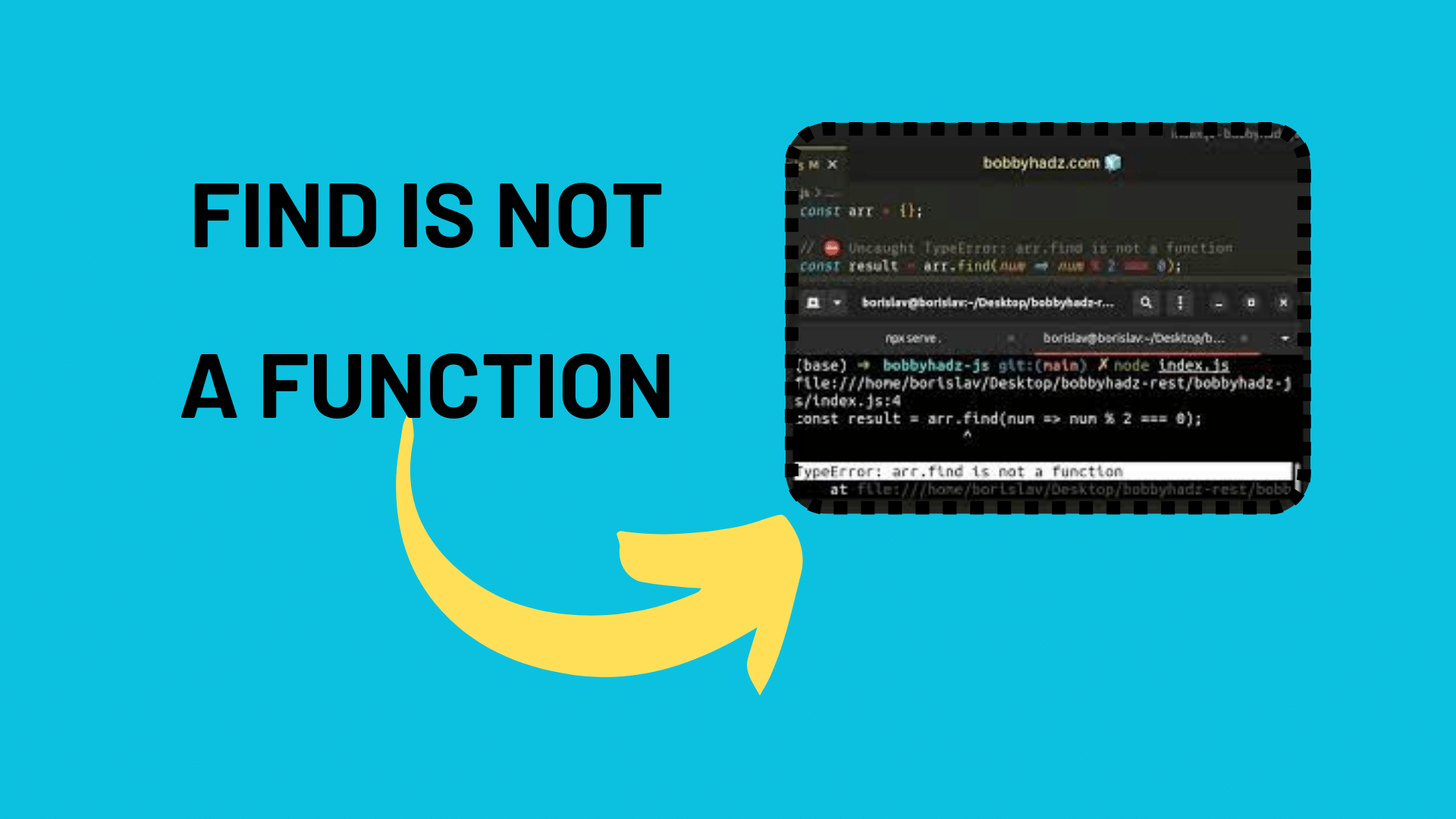
How to Resolving Script Issues: Fixing the ‘Find is not a Function’ Error
JavaScript is a powerful and versatile programming language, but sometimes even experienced developers encounter errors that can be puzzling. In this tutorial, we will address the common “Stations.find is not a function” error and provide a solution to resolve it.
Error Scenario:
Imagine you have a JavaScript project where you need to search for a specific value in an array stored in another file. You attempt to use the find
method to locate the desired value, but unexpectedly, you encounter the error message: “Stations.find is not a function.” Let’s dive into the details of this issue and find out how to fix it.
Problem Description:
You have a variable called myStation
containing a value, and you want to find this value in an array located in a separate file named station.js
. Your goal is to retrieve the stationID
associated with the matching station name. Below is a snippet of the code that leads to the error:
const Stations = require("./stations.js");
const myStation = handlerInput.requestEnvelope.request.intent.slots.stationName.value;
let stationNewName = Stations.find((s) => s.stationName === myStation);
You want to understand what is causing this error and how to modify your code to resolve it.
Analysis:
The error message, “Stations.find is not a function,” suggests that the find
method is not available on the Stations
object as you expected. This issue is likely due to the structure of your exported module in stations.js
. Let’s examine it:
module.exports = {
STATIONS: [
{stationName: "CBS", stationID: "8532885"},
{stationName: "NBC", stationID: "8533935"},
{stationName: "ABC", stationID: "8534048"},
],
};
Here, you are exporting an object with a property named STATIONS
, which contains your array of station data.
Solution:
To resolve the error, you should reference the STATIONS
property when using the find
method. Update your code as follows:
const Stations = require("./stations.js");
const myStation = handlerInput.requestEnvelope.request.intent.slots.stationName.value;
let stationNewName = Stations.STATIONS.find((s) => s.stationName === myStation);
By accessing the STATIONS
property of the Stations
object, you correctly target the array and can use the find
method to search for the desired station.
Conclusion:
In this tutorial, we addressed the “Stations.find is not a function” error in JavaScript. The key to resolving this error was understanding the structure of the exported module and referencing the correct property containing the array. By following the provided solution, you can successfully find the station and retrieve its stationID
without encountering any errors in your code.
[…] Fixing the ‘Find is not a Function’ Error in Your Script […]
[…] Fixing the ‘Find is not a Function’ Error in Your Script […]