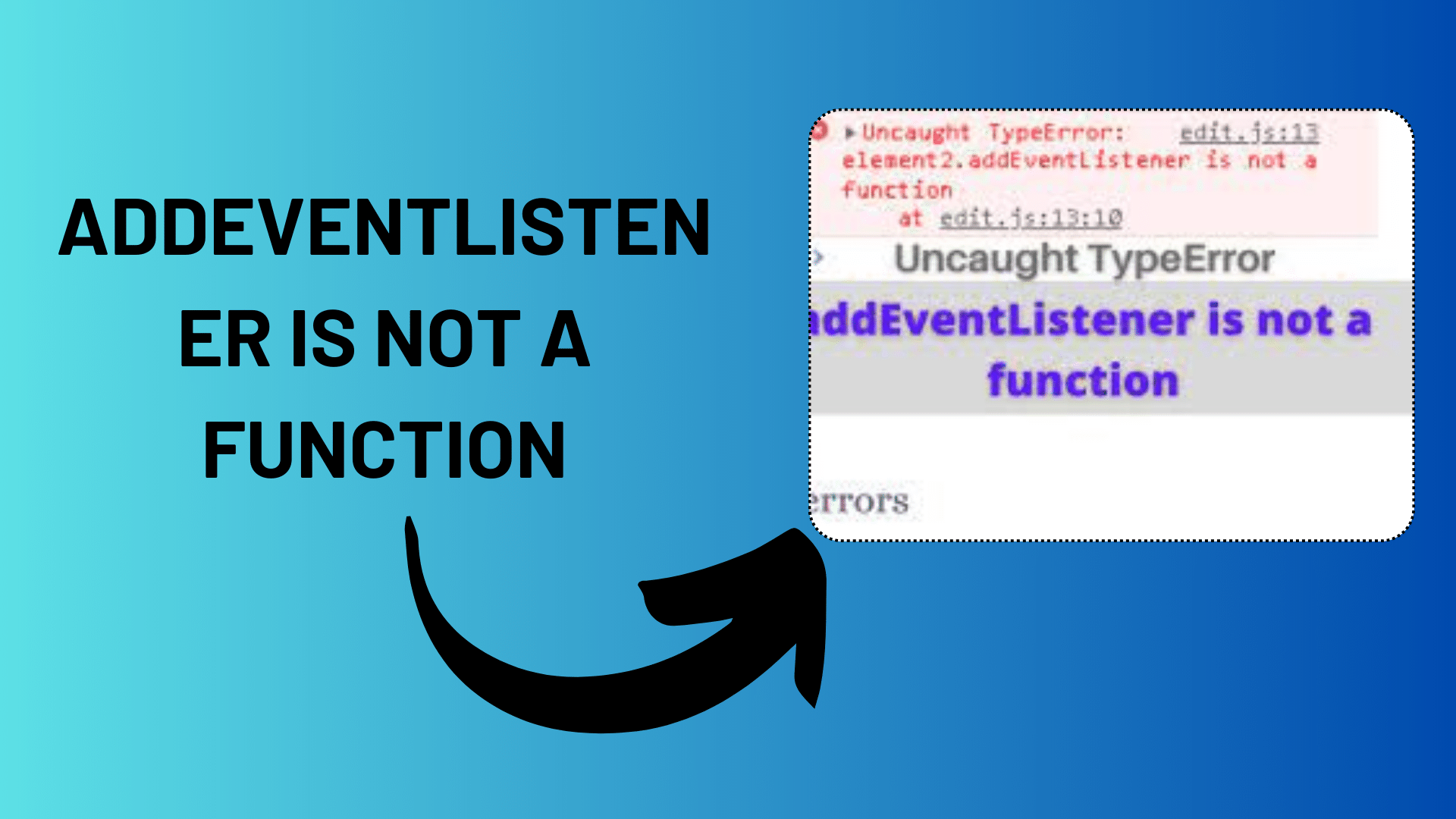
How to Solve JavaScript ‘addEventListener is not a Function’ Error
If you’ve encountered the “.addEventListener is not a function” error in your JavaScript code, you’re not alone. This error is a common issue that often occurs when working with event listeners in JavaScript. In this blog post, we’ll explore the possible reasons behind this error and provide solutions to help you resolve it.
What Does the Error Mean?
The error message “.addEventListener is not a function” indicates that you are trying to use the addEventListener
method on an object that doesn’t support it. In most cases, this happens when you mistakenly apply the addEventListener
method to an array-like object instead of an individual element.
Understanding Your Code
Let’s take a look at the code snippet that triggered this error:
var comment = document.getElementsByClassName("button");
function showComment() {
var place = document.getElementById('textfield');
var commentBox = document.createElement('textarea');
place.appendChild(commentBox);
}
comment.addEventListener('click', showComment, false);
In this code, you’re trying to add a click event listener to elements with the class name “button.” Here’s what’s happening:
- You use
document.getElementsByClassName("button")
to select all elements with the class name “button.” This returns an array-like object of elements. - You define a
showComment
function that creates a textarea element and appends it to an element with the id “textfield.” - Finally, you attempt to add a click event listener to the
comment
object, which is an array-like object.
Solutions to the Error
To fix the “.addEventListener is not a function” error in your code, you have a few options:
1. Target a Specific Element
If you want to add an event listener to a specific element, you should target that element directly using its ID or another unique identifier. Here’s an example:
var comment = document.getElementById("button"); // Use the element's ID
comment.addEventListener('click', showComment, false);
2. Loop Through Elements
If you intend to add event listeners to multiple elements with the same class name, you should loop through the array-like object returned by getElementsByClassName
and add the event listener to each element individually. Here’s how you can do it:
var comments = document.getElementsByClassName("button");
function showComment() {
var place = document.getElementById('textfield');
var commentBox = document.createElement('textarea');
place.appendChild(commentBox);
}
for (var i = 0; i < comments.length; i++) {
comments[i].addEventListener('click', showComment, false);
}
In this example, we iterate through each element with the class name “button” and add the click event listener to each one.
3. Use querySelector Instead
Another approach is to use document.querySelector
to target the element you want directly. This method returns the first matching element, which can be helpful if you only need to select one element with a specific class name or ID. Here’s an example:
var comment = document.querySelector(".button"); // Select the first matching element
comment.addEventListener('click', showComment, false);
Conclusion
The “.addEventListener is not a function” error is a common issue when working with event listeners in JavaScript. It typically occurs when you try to use the addEventListener
method on an array-like object instead of an individual element. To resolve this error, make sure to target the correct element and consider looping through elements if you need to add event listeners to multiple elements with the same class name. Understanding how to use event listeners effectively will help you build interactive and responsive web applications.
[…] Fixing ‘addeventlistener is not a function’ in Your Code […]