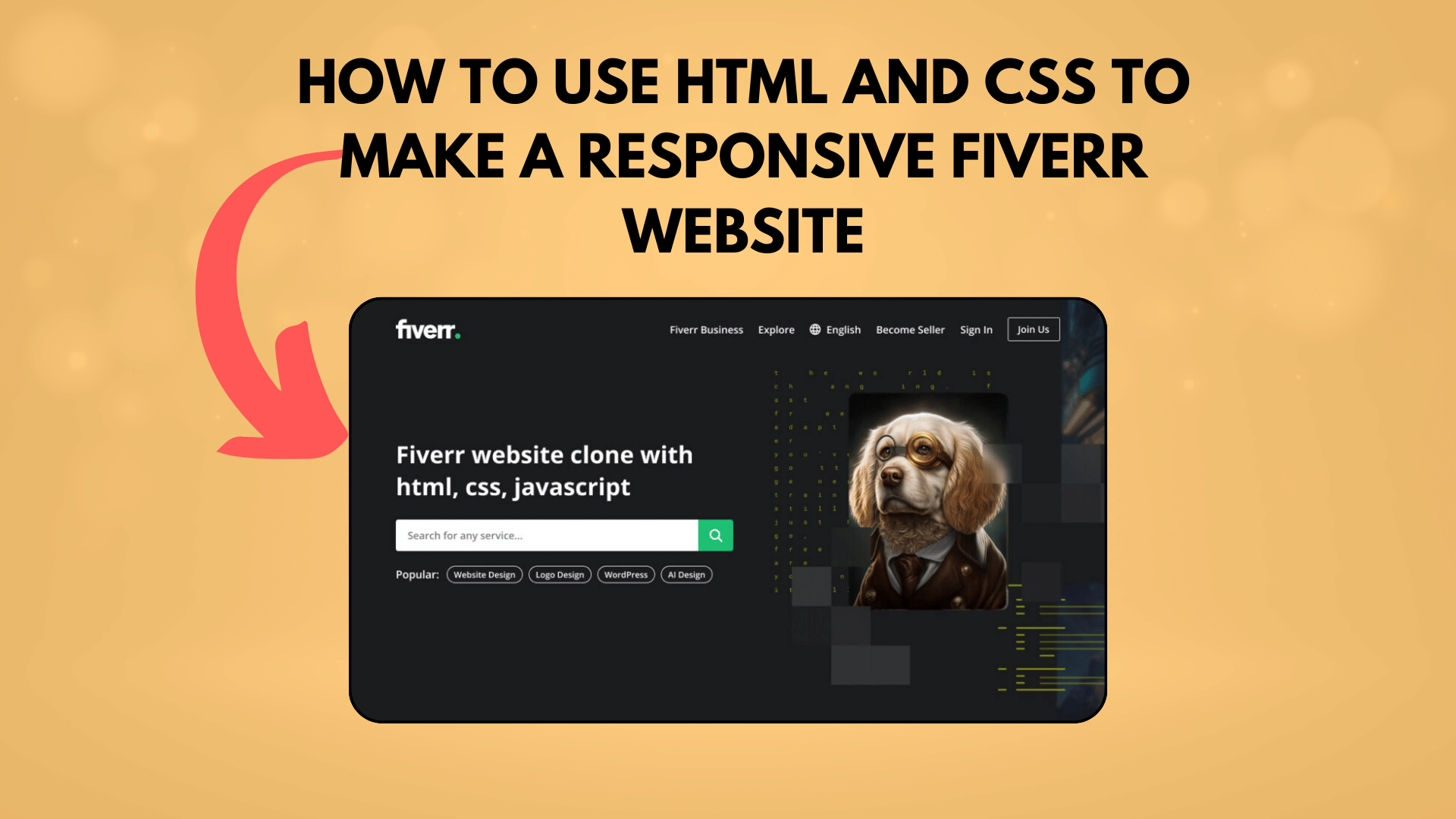
How to Use HTML and CSS to Make a Responsive Fiverr Website
Making a Fiverr website using HTML and CSS is a great way for a novice web developer to get practical expertise. You may show off your abilities to copy current designs in addition to honing your practical HTML and CSS skills by developing the design of a reputable website like Fiverr.
I’ll walk you through the process of making a responsive Fiverr website using just HTML, CSS, and JavaScript in this simple blog article. You’ll discover how to design an interactive homepage for a website that includes a navigation bar, arrange objects on the page, and style them to blend in with the Fiverr aesthetic.
We’ll examine a range of HTML elements and CSS features in this tutorial to effectively design our site, which draws inspiration from Fiverr. We’ll utilize tags like nav, sections, divs, inputs, links, and other frequently used elements to construct an eye-catching layout that draws users in and guarantees a straightforward interface.
HTML and CSS Video Tutorial for the Fiverr Website
Steps To Create Fiverr Website in HTML and CSS
To create a responsive Fiverr-inspired Homepage using HTML and CSS, follow these step-by-step instructions:
- First, create a folder with any name you like. Then, put the necessary files inside it.
- Create a file called
index.html
to serve as the main file. - Create a file called
style.css
for the CSS code. - Download and place the Images folder in your project directory. This folder includes the Fiverr logo and the hero background image.
To start, add the following HTML codes to your index.html
file. These codes include a navigation bar (nav), sections, input fields, links, and various other tags necessary for your webpage. Additionally, this code includes a few lines of JavaScript code to toggle the mobile menu on small screens.
<!DOCTYPE html>
<!-- Coding By CodingNepal - www.codingnepalweb.com -->
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Fiverr Homepage Clone | CodingNepal</title>
<link rel="stylesheet" href="style.css">
<!-- Google Icons Link -->
<link rel="stylesheet" href="https://fonts.googleapis.com/css2?family=Material+Symbols+Outlined:opsz,wght,FILL,GRAD@48,400,0,0">
</head>
<body>
<header>
<nav class="navbar">
<a href="#" class="logo">
<img src="images/logo.svg" alt="Fiverr Logo">
</a>
<ul class="menu-links">
<li><a href="#">Fiverr Business</a></li>
<li><a href="#">Explore</a></li>
<li class="language-item">
<a href="#">
<span class="material-symbols-outlined">language</span>
English
</a>
</li>
<li><a href="#">Become a Seller</a></li>
<li><a href="#">Sign In</a></li>
<li class="join-btn"><a href="#">Join Us</a></li>
<span id="close-menu-btn" class="material-symbols-outlined">close</span>
</ul>
<span id="hamburger-btn" class="material-symbols-outlined">menu</span>
</nav>
</header>
<section class="hero-section">
<div class="content">
<h1>Find the right freelance service, right away</h1>
<form action="#" class="search-form">
<input type="text" placeholder="Search for any service..." required>
<button class="material-symbols-outlined" type="sumbit">search</button>
</form>
<div class="popular-tags">
Popular:
<ul class="tags">
<li><a href="#">Webite Design</a></li>
<li><a href="#">Logo Design</a></li>
<li><a href="#">WordPress</a></li>
<li><a href="#">AI Design</a></li>
</ul>
</div>
</div>
</section>
<script>
const header = document.querySelector("header");
const hamburgerBtn = document.querySelector("#hamburger-btn");
const closeMenuBtn = document.querySelector("#close-menu-btn");
// Toggle mobile menu on hamburger button click
hamburgerBtn.addEventListener("click", () => header.classList.toggle("show-mobile-menu"));
// Close mobile menu on close button click
closeMenuBtn.addEventListener("click", () => hamburgerBtn.click());
</script>
</body>
</html>
Next, update your style with the following CSS codes.css file to replicate the Fiverr homepage on your website. Different styles for elements like as color, border, background, and even the homepage picture are included in these scripts. They also use media queries to make your webpage responsive.
/* Importing Google font - Fira Sans */
@import url('https://fonts.googleapis.com/css2?family=Fira+Sans:wght@300;400;500;600;700&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Fira Sans', sans-serif;
}
body {
background: #1B1B1D;
}
header {
position: fixed;
left: 0;
top: 0;
width: 100%;
z-index: 1;
padding: 20px;
}
header .navbar {
max-width: 1280px;
margin: 0 auto;
display: flex;
align-items: center;
justify-content: space-between;
}
.navbar .menu-links {
display: flex;
align-items: center;
list-style: none;
gap: 30px;
}
.navbar .menu-links li a {
color: #fff;
font-weight: 500;
text-decoration: none;
transition: 0.2s ease;
}
.navbar .menu-links .language-item a {
display: flex;
gap: 8px;
align-items: center;
}
.navbar .menu-links .language-item span {
font-size: 1.3rem;
}
.navbar .menu-links li a:hover {
color: #1dbf73;
}
.navbar .menu-links .join-btn a {
border: 1px solid #fff;
padding: 8px 15px;
border-radius: 4px;
}
.navbar .menu-links .join-btn a:hover {
color: #fff;
border-color: transparent;
background: #1dbf73;
}
.hero-section {
height: 100vh;
background-image: url("images/hero-img.jpg");
background-position: center;
background-size: cover;
position: relative;
display: flex;
padding: 0 20px;
align-items: center;
}
.hero-section .content {
max-width: 1280px;
margin: 0 auto 40px;
width: 100%;
}
.hero-section .content h1 {
color: #fff;
font-size: 3rem;
max-width: 630px;
line-height: 65px;
}
.hero-section .search-form {
height: 48px;
display: flex;
max-width: 630px;
margin-top: 30px;
}
.hero-section .search-form input {
height: 100%;
width: 100%;
border: none;
outline: none;
padding: 0 15px;
font-size: 1rem;
border-radius: 4px 0 0 4px;
}
.hero-section .search-form button {
height: 100%;
width: 60px;
border: none;
outline: none;
cursor: pointer;
background: #1dbf73;
color: #fff;
border-radius: 0 4px 4px 0;
transition: background 0.2s ease;
}
.hero-section .search-form button:hover {
background: #19a463;
}
.hero-section .popular-tags {
display: flex;
color: #fff;
gap: 25px;
font-size: 0.875rem;
font-weight: 500;
margin-top: 25px;
}
.hero-section .popular-tags .tags {
display: flex;
gap: 15px;
align-items: center;
list-style: none;
}
.hero-section .tags li a {
text-decoration: none;
color: #fff;
border: 1px solid #fff;
padding: 4px 12px;
border-radius: 50px;
transition: 0.2s ease;
}
.hero-section .tags li a:hover {
color: #000;
background: #fff;
}
.navbar #hamburger-btn {
color: #fff;
cursor: pointer;
display: none;
font-size: 1.7rem;
}
.navbar #close-menu-btn {
position: absolute;
display: none;
color: #000;
top: 20px;
right: 20px;
cursor: pointer;
font-size: 1.7rem;
}
@media screen and (max-width: 900px) {
header.show-mobile-menu::before {
content: "";
height: 100%;
width: 100%;
position: fixed;
left: 0;
top: 0;
backdrop-filter: blur(5px);
}
.navbar .menu-links {
height: 100vh;
max-width: 300px;
width: 100%;
background: #fff;
position: fixed;
left: -300px;
top: 0;
display: block;
padding: 75px 40px 0;
transition: left 0.2s ease;
}
header.show-mobile-menu .navbar .menu-links {
left: 0;
}
.navbar .menu-links li {
margin-bottom: 30px;
}
.navbar .menu-links li a {
color: #000;
font-size: 1.1rem;
}
.navbar .menu-links .join-btn a {
padding: 0;
}
.navbar .menu-links .join-btn a:hover {
color: #1dbf73;
background: none;
}
.navbar :is(#close-menu-btn, #hamburger-btn) {
display: block;
}
.hero-section {
background: none;
}
.hero-section .content {
margin: 0 auto 80px;
}
.hero-section .content :is(h1, .search-form) {
max-width: 100%;
}
.hero-section .content h1 {
text-align: center;
font-size: 2.5rem;
line-height: 55px;
}
.hero-section .search-form {
display: block;
margin-top: 20px;
}
.hero-section .search-form input {
border-radius: 4px;
}
.hero-section .search-form button {
margin-top: 10px;
border-radius: 4px;
width: 100%;
}
.hero-section .popular-tags {
display: none;
}
}
Conclusion and Final words
In conclusion, learning HTML, CSS, and JavaScript to create a Fiverr website is a useful assignment, especially for inexperienced web programmers. You’ve successfully made a responsive homepage inspired by Fiverr by following the steps listed in this tutorial.
Don’t forget to continue playing with code and developing your abilities by building increasingly stunning webpages. I advise looking over and copying some of the other amazing website designs that are accessible on this page.
By selecting the Download option, you can get the source code files for this Fiverr Homepage project for free in case you run into any issues when building your Fiverr website. By selecting the View Live option, you can also see a live demonstration of it.
[…] touch of JavaScript. Earlier, I have created various blogs of Responsive Navigation Bar using HTML and CSS, but I haven’t built any drop-down navigation menu […]