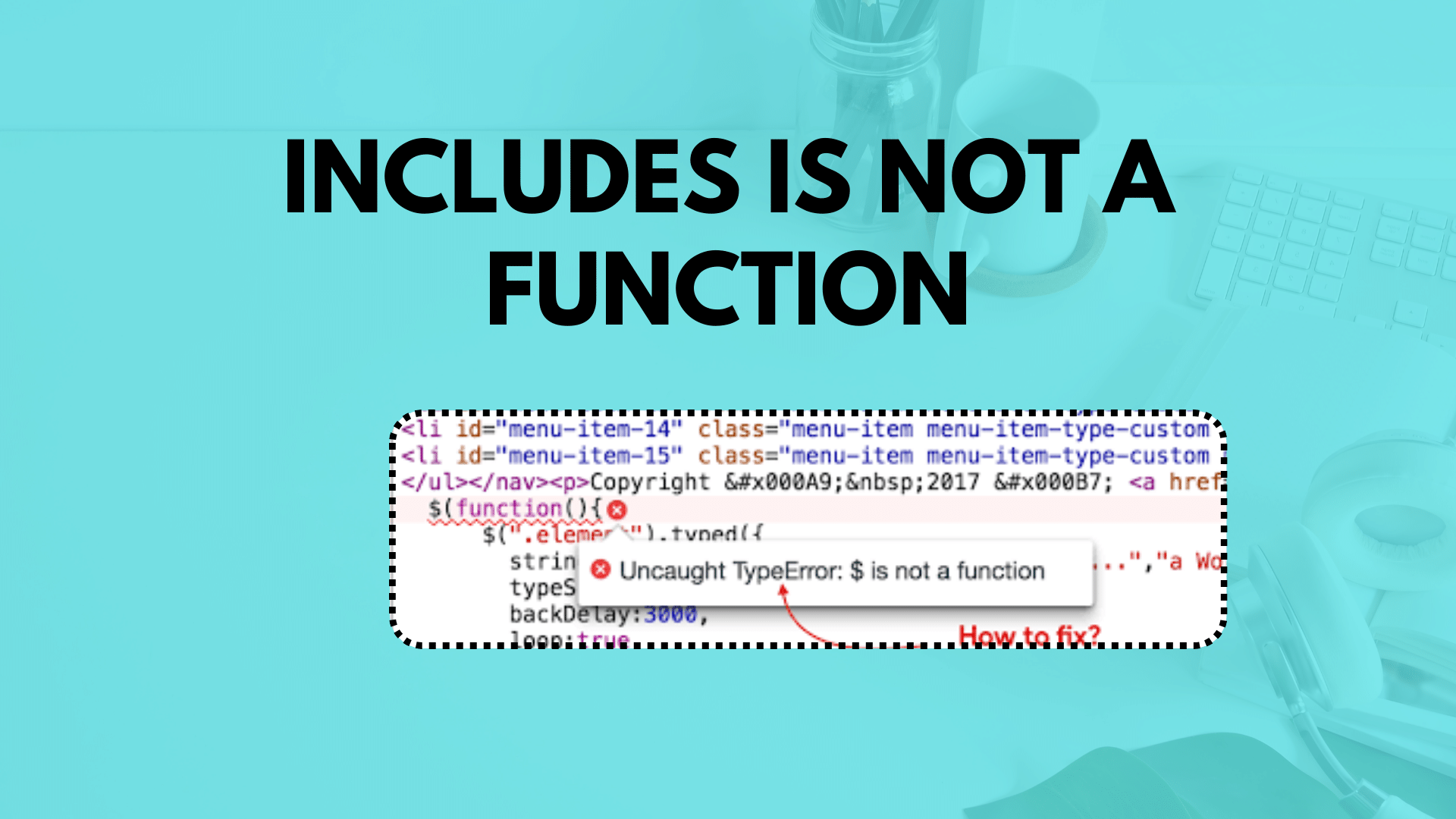
How to Fix ‘Includes is not a function’ Errors
JavaScript is a versatile and powerful programming language, but it’s not immune to errors. One common error that you may encounter is the “TypeError: includes is not a function” error. This error occurs when the includes()
method is called on a value that is not of type string or array. In this article, we will explore this error in detail and discuss how to solve it.
Understanding the Error:
Let’s start by understanding what causes this error. Consider the following code snippet:
const str = 1234;
// ⛔️ Uncaught TypeError: str.includes is not a function
const result = str.includes('3');

In this example, we attempted to use the includes()
method on a numeric value (1234
). However, the includes()
method is only available on strings and arrays, not on numbers. As a result, JavaScript throws a “TypeError: includes is not a function” error.
Solutions to the Error:
To resolve this error, we have a few options:
- Convert the Value to a String or Array: One way to fix the error is to ensure that the value is of the correct type (string or array) before using the
includes()
method. Here are two examples of how to do this:
// ✅ Convert to a String before using includes()
const num = 1234;
const result1 = String(num).includes('3');
console.log(result1); // 👉️ true
In this example, we used the String()
constructor to convert the numeric value num
into a string before using includes()
.
// ✅ Convert to an Array before using includes()
const set = new Set(['a', 'b', 'c']);
const result2 = Array.from(set).includes('b');
console.log(result2); // 👉️ true
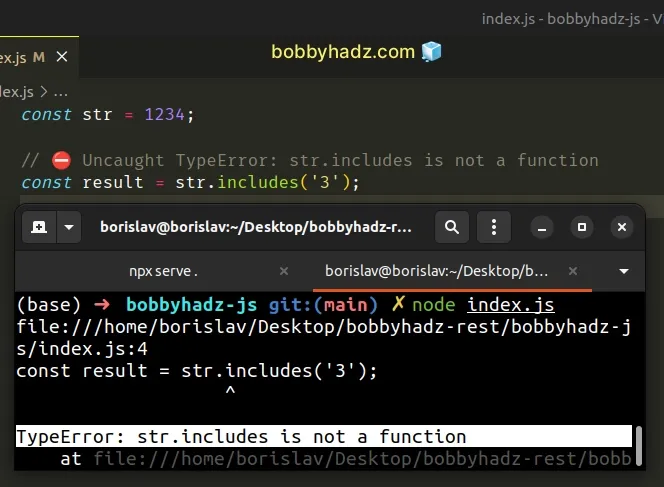
Here, we converted a Set into an array using Array.from()
and then used includes()
on the array.
- Conditionally Check the Value’s Type: Another approach is to conditionally check if the value is of the correct type before calling the
includes()
method. This can be done using the ternary operator or a simpleif
statement:
// ✅ Check if the value is a String before using includes()
const num = 1234;
const result1 = typeof num === 'string' ? num.includes('3') : false;
console.log(result1); // 👉️ false
In this example, we check if num
is of type string before attempting to use includes()
. If it’s not a string, we return false
.
// ✅ Check if the value is an Array before using includes()
const set = new Set(['a', 'b', 'c']);
const result2 = Array.isArray(set) ? set.includes('b') : false;
console.log(result2); // 👉️ false
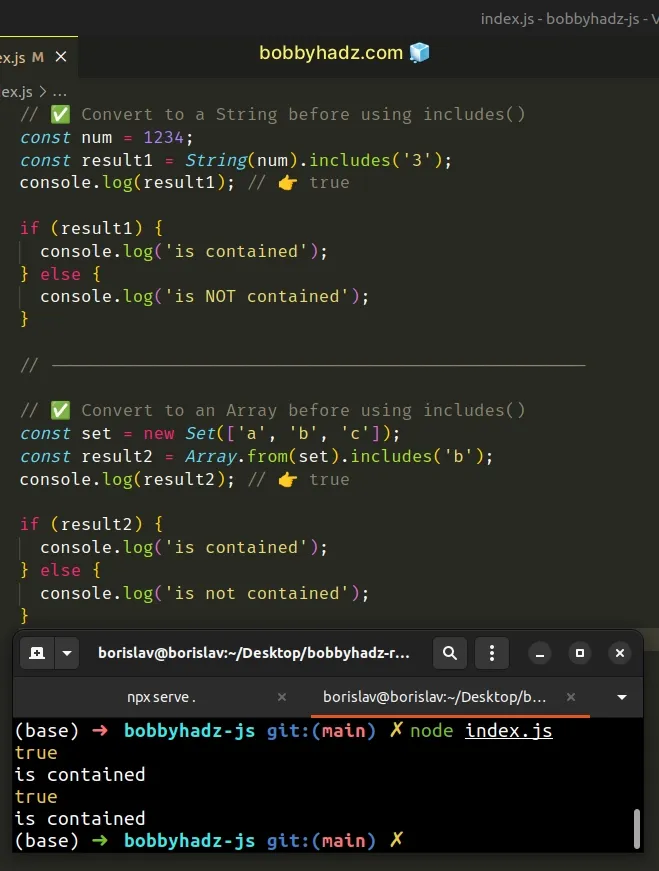
Here, we use Array.isArray()
to check if set
is an array before calling includes()
.
- Access Specific Property on Objects: If you are working with objects, you may need to access a specific property that contains a string or an array value before using
includes()
. For example:
const obj = {
site: ['bobby', 'hadz', 'com'],
};
console.log(obj.site.includes('bobby')); // 👉️ true
In this case, we access the site
property of the obj
object, which is an array, before calling includes()
.
Conclusion:
The “TypeError: includes is not a function” error is a common issue in JavaScript, but it can be easily resolved by ensuring that you’re calling the includes()
method on the appropriate data type (string or array). Whether you choose to convert the value, conditionally check its type, or access specific properties, these solutions will help you avoid this error and make your code more robust. Happy coding!