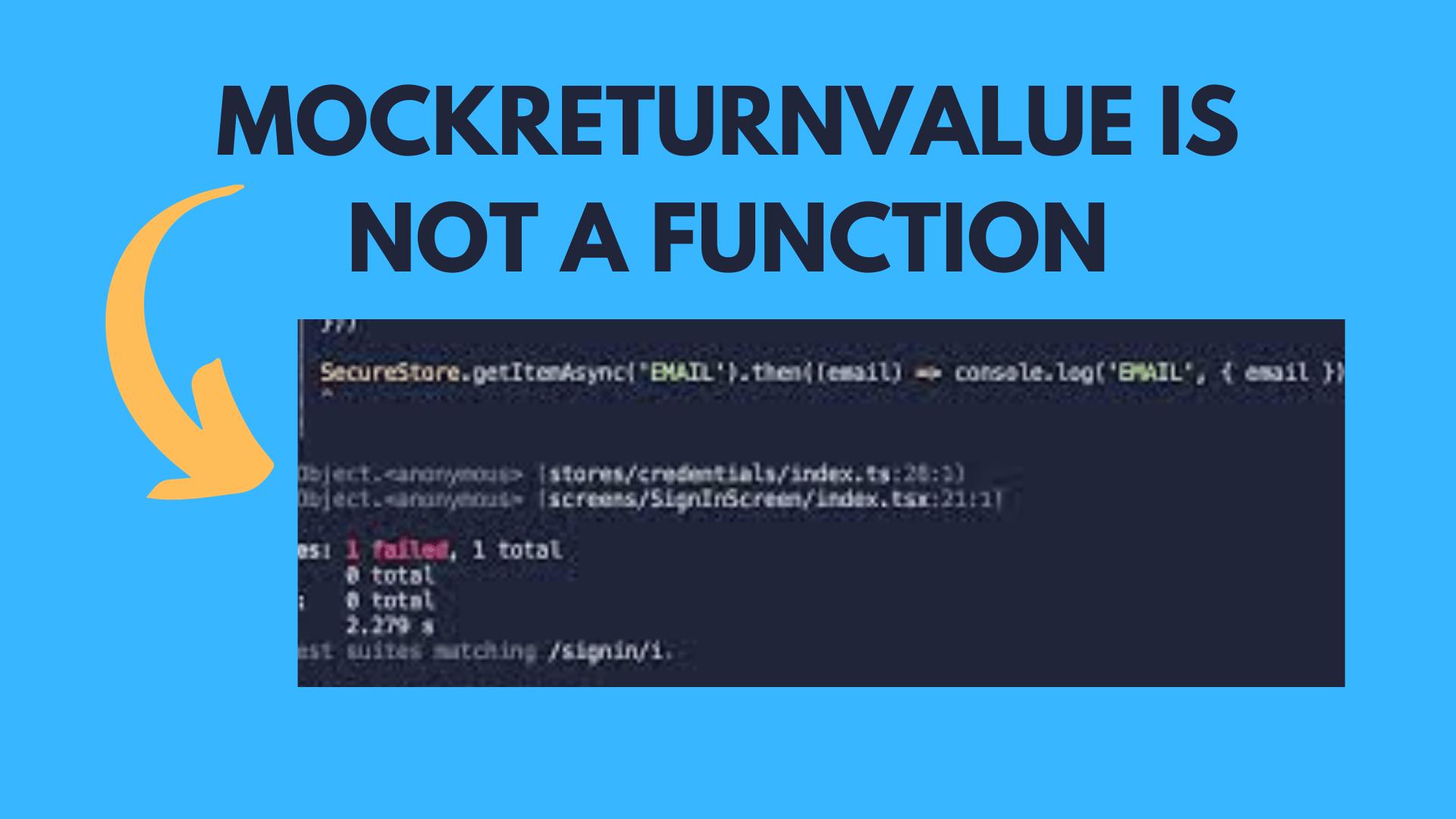
How to Resolve ‘MockReturnValue is not a Function’ Errors in Unit Testing
In the world of unit testing with Jest, encountering errors can be a common occurrence. One such error that developers often face is the “.mockReturnValue is not a function” error. This error can be particularly frustrating when you’re trying to mock functions and test your code effectively. In this article, we’ll explore a real-world scenario where this error occurs and discuss how to troubleshoot and resolve it.
The Scenario:
Let’s dive into a practical scenario to better understand the issue. Imagine you have a TypeScript module named module.ts
with multiple named exports:
// module.ts
export function someFunctionOne() {
// implementation
}
export const someFunctionTwo = function () {
// implementation
}
export const someFunctionThree = () => {
// implementation
}
export const someFunctionFour = () => {
// implementation
}
In your unit tests, you need to mock this module in various ways. Some exports should be initially mocked to specific values, while others need different mocks for specific tests.
Your test file, module.test.ts
, might look something like this:
import React from 'react'
import { shallow } from 'enzyme'
import Component from './component' // component under test
import { someFunctionOne, someFunctionTwo } from './module'
;(jest as any).mock('./module', () => ({
someFunctionOne: jest.fn(),
someFunctionTwo: jest.fn(),
someFunctionThree: jest.fn(() => 10),
someFunctionFour: jest.fn((s) => s),
}))
;(someFunctionOne as any).mockReturnValue('some String')
;(someFunctionTwo as any).mockReturnValue(false)
The Error:
Now, here’s where the problem arises. When you try to mock the functions using .mockReturnValue
or similar methods, you encounter the dreaded “.mockReturnValue is not a function” error:
TypeError: _moduleName.someFunctionOne.mockReturnValue is not a function
This error can be frustrating and confusing, especially when you’ve followed what seems to be the correct mocking process.
Troubleshooting the Error:
Let’s explore some troubleshooting steps to resolve this error and get your tests running smoothly.
- Check jest.setup.js: Ensure that you’ve added the necessary module mocking in your
jest.setup.js
file or equivalent setup file. This is where you should set up your module mocks. Double-check if the module is correctly mocked there. - Hoisting Issues: Sometimes, Jest mocking might not work as expected due to hoisting problems. To address this, consider changing your import statements to require statements and ensure that they appear below the
jest.mock
statement in your test file. - __esModule Property: While working with named exports in ES6 modules, ensure that you include
__esModule: true
in your module mock. This property can affect how named exports are handled in your tests. - Jest and Babel Configuration: Review your Jest and Babel configuration to make sure there are no misconfigurations or conflicts that could interfere with module mocking.
Conclusion:
The “.mockReturnValue is not a function” error can be a challenging obstacle when trying to write effective unit tests with Jest. By following the troubleshooting steps mentioned above, you can identify and resolve the underlying issues in your testing environment. Remember to double-check your setup, be mindful of hoisting, and ensure that your module mocks are correctly configured. With these steps, you’ll be better equipped to tackle this error and continue writing reliable unit tests for your codebase.
In the world of software development, testing is a crucial aspect of ensuring the quality and reliability of your applications. Identifying and resolving errors like this one is a valuable skill that can enhance your testing capabilities and contribute to the overall success of your projects. Happy coding and testing!
[…] How to Resolve ‘MockReturnValue is not a Function’ Errors in Unit Testing […]