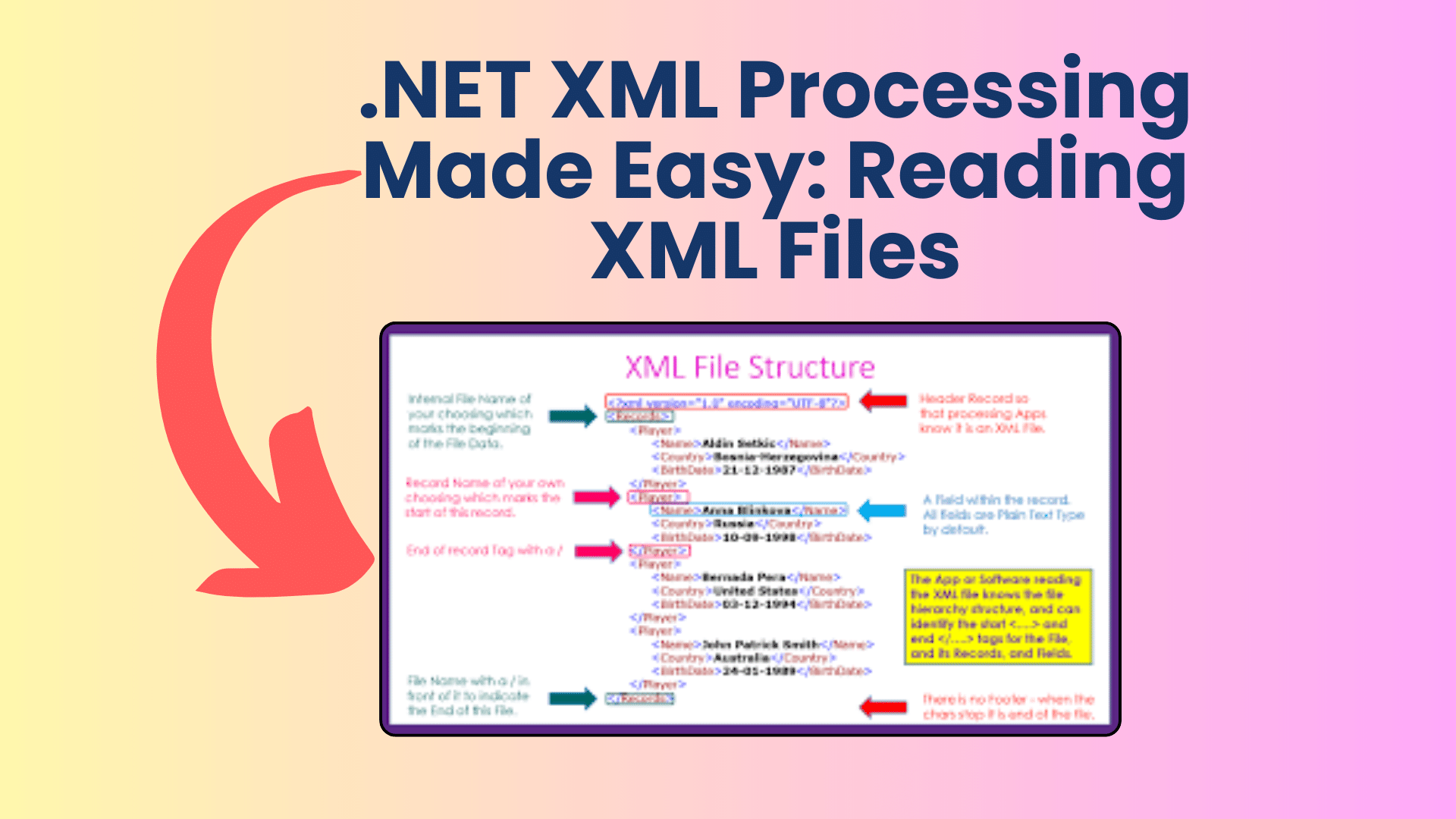
.NET XML Processing Made Easy: Reading XML Files
When it comes to loading and parsing XML files in a .NET application, it’s essential to find a straightforward and efficient way to access the data you need. In this tutorial, we’ll explore how you can make XML processing easy in .NET, focusing on reading XML files. We’ll use a sample XML structure that contains program and user settings as an example to demonstrate the process.
XML File Structure: First, let’s take a look at the structure of the XML file we’ll be working with. This XML file contains two main sections: “ProgramSettings” and “UserSettings.” It’s designed to store configuration settings for an application, including database file paths, user-specific layout preferences, and data file locations.
You Might Like This :
- How to Handle “.NET Location Not Found” in Your .NET Projects
- Convert HTML to PDF in .NET: A Comprehensive Guide
- What You Need to Know About the .NET Framework 4.8 End of Life
- A Guide to Background Processing in .NET MAUI Apps
<ProgramSettings>
<database file="C:\database.mdb" />
</ProgramSettings>
<UserSettings>
<user key="user1">
<layout color="red" fontsize="5" />
<data file="C:\test1.txt" />
</user>
<user key="user2">
<layout color="blue" fontsize="2" />
<data file="C:\test2.txt" />
</user>
</UserSettings>
As shown in the XML structure above, “ProgramSettings” contain global configuration data, while “UserSettings” store individual user preferences.
Reading XML Using LINQ to XML: One efficient way to read XML files in .NET is by using LINQ to XML. It allows you to query and manipulate XML data easily. Here’s how you can read values from the XML structure mentioned above:
using System;
using System.Linq;
using System.Xml.Linq;
// Load the XML file
XDocument xmlDoc = XDocument.Load("your_xml_file.xml");
string userLogin = "user1";
// Query for the color and font size of a specific user
XElement userElement = xmlDoc.Element("UserSettings")
.Elements("user")
.FirstOrDefault(u => u.Attribute("key").Value == userLogin);
if (userElement != null)
{
string color = userElement.Element("layout").Attribute("color").Value;
string fontSize = userElement.Element("layout").Attribute("fontsize").Value;
Console.WriteLine($"User {userLogin} preferences - Color: {color}, Font Size: {fontSize}");
}
In the code above, we load the XML file into an XDocument and then use LINQ to XML to query for the user’s settings based on the “userLogin” variable.
Modifying XML Values: If you want to edit values in the XML file, you can do so with LINQ to XML as well. Here’s how you can change the font size for a specific user:
// Modify the font size for a user
userElement.Element("layout").SetAttributeValue("fontsize", "green");
// Save the changes back to the XML file
xmlDoc.Save("your_xml_file.xml");
Conclusion:
In this tutorial, we’ve demonstrated how to make XML processing easy in .NET by reading XML files using LINQ to XML. This approach allows you to access and modify XML data efficiently, making it a powerful tool for managing configuration settings or any other XML-based data in your .NET applications.
[…] .NET XML Processing Made Easy: Reading XML Files […]
[…] NET XML Processing Made Easy: Reading XML Files […]
I’m extremely impressed with your writing siills andd also with the laqyout on your blog.
Is this a paid theme or did you modify it yourself? Either way keep up the
nice quality writing, it’s rare to see a great blog like this one nowadays.
my web page – Kalol call girl