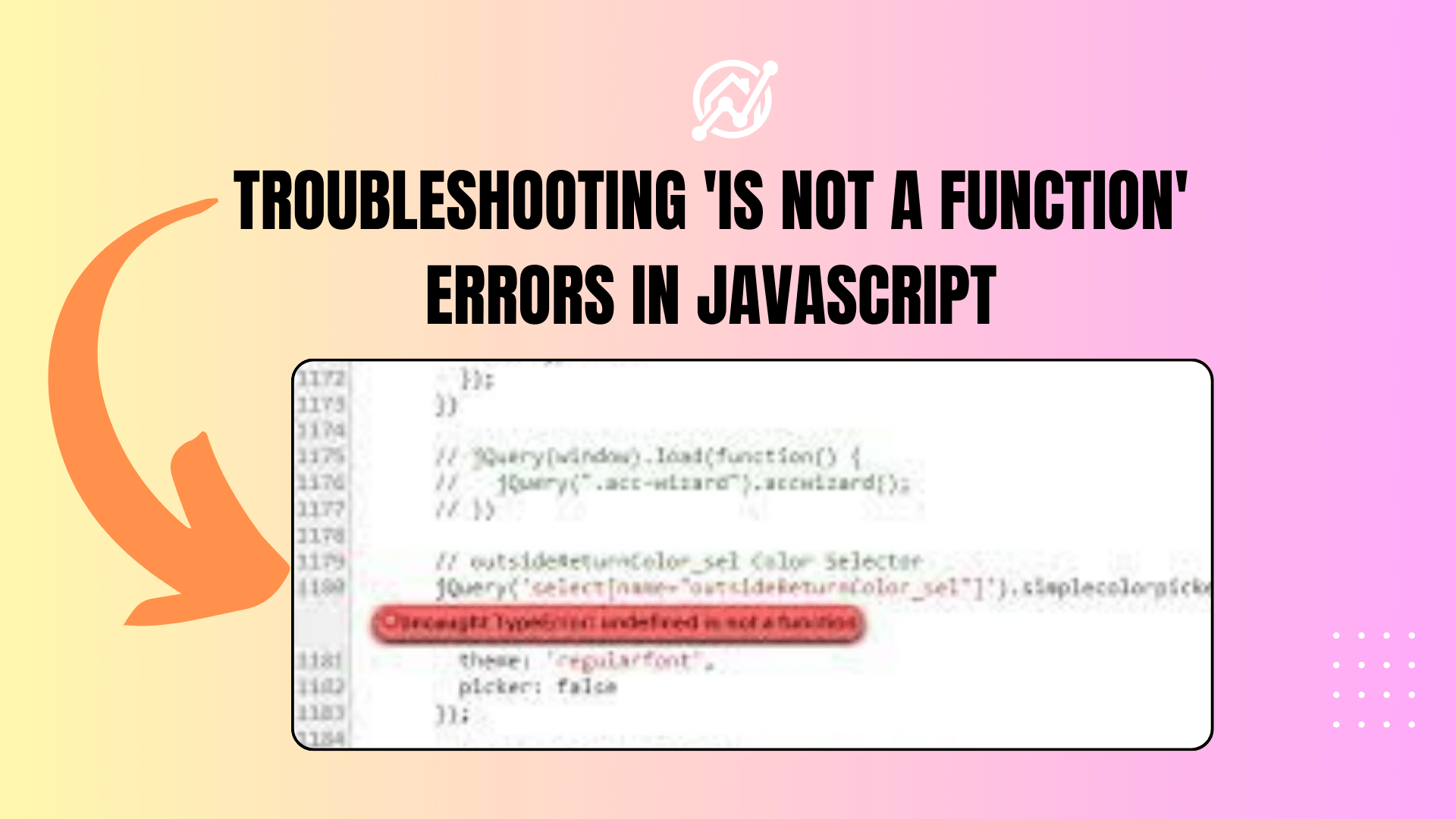
Ho To Troubleshooting ‘is not a function’ errors in JavaScript
JavaScript is a versatile and powerful programming language used extensively in web development. One common task in JavaScript is splitting strings into arrays using the .split()
function. However, you may have encountered an error message like this:
“Uncaught TypeError: string.split is not a function”
In this blog post, we will explore why you might encounter this error and how to prevent it. We will delve into the intricacies of JavaScript’s data types and provide practical solutions to this common pitfall.
Understanding the Error:
Let’s start by understanding why you get this error. The error message occurs when you try to use the .split()
function on a variable that is not a string. In the provided code snippet:
var string = document.location;
var split = string.split('/');
document.location
is an object, not a string. This is where the problem begins. To avoid this error, you need to ensure that you are working with a string when you call .split()
.
You Might Like This :
- JavaScript Cookie Management: Resolving the “Cookie is not a function” Error
- How to Perform Mass Replacements in JavaScript
- Solving Form Validation Challenges with HTML, CSS, and JavaScript
- How to Resolve ‘MockReturnValue is not a Function’ Errors in Unit Testing
Solutions:
1.Converting to String:
One straightforward solution is to explicitly convert the object to a string. You can achieve this by appending an empty string to the object:
var string = document.location + '';
var split = string.split('/');
This works because JavaScript will call the .toString()
method of the object, providing a string representation.
Alternatively, you can use document.location.href
or document.location.pathname
directly, which are strings:
2.var string = document.location.href;
var split = string.split('/');
2.Using .toString()
Method:
Another option is to use the .toString()
method explicitly:
var string = document.location.toString();
var split = string.split('/');
This approach is more readable and avoids any potential confusion
3. Check the Variable Type:To prevent this error from occurring in the first place, you can check the variable’s type before using .split()
. For example:
var string = document.location;
if (typeof string === 'string') {
var split = string.split('/');
} else {
// Handle the case where 'string' is not a string.
}
Conclusion:
The “Uncaught TypeError: string.split is not a function” error in JavaScript is a common issue when working with the .split()
function. It usually occurs when trying to split a non-string variable. To prevent this error, make sure you are working with a string or convert the variable to a string using one of the methods mentioned above. Additionally, consider checking the variable’s type before applying string-specific functions to avoid such pitfalls in your JavaScript code.
By following these guidelines, you can write more robust JavaScript code and minimize errors related to the .split()
function. Happy coding!
[…] Troubleshooting ‘is not a function’ errors in JavaScript […]